Calling a Class Method from a Trigger
You can call public utility methods from a trigger. Calling methods of other classes enables code reuse, reduce the size of your triggers, and improves maintenance of your Apex code. It also allows you to use object-oriented programming.
In this example, we will call a static method from a trigger. When the trigger is fired because of an insert event, our code will call our method sendMail() from the class of EmailManager.
Here is the EmailManager class that we will use for our example.
public class EmailManager {
// Public method
public static void sendMail(String address, String subject, String body) {
// Create an email message object
Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage();
String[] toAddresses = new String[] {address};
mail.setToAddresses(toAddresses);
mail.setSubject(subject);
mail.setPlainTextBody(body);
// Pass this email message to the built-in sendEmail method
// of the Messaging class
Messaging.SendEmailResult[] results = Messaging.sendEmail(
new Messaging.SingleEmailMessage[] { mail });
// Call a helper method to inspect the returned results
inspectResults(results);
}
// Helper method
private static Boolean inspectResults(Messaging.SendEmailResult[] results) {
Boolean sendResult = true;
// sendEmail returns an array of result objects.
// Iterate through the list to inspect results.
// In this class, the methods send only one email,
// so we should have only one result.
for (Messaging.SendEmailResult res : results) {
if (res.isSuccess()) {
System.debug('Email sent successfully');
}
else {
sendResult = false;
System.debug('The following errors occurred: ' + res.getErrors());
}
}
return sendResult;
}
}
The above class basically sends an email when it is used. We are going to use it inside of our trigger.
Here is our trigger.
trigger ExampleTrigger on Contact (after insert, after delete) {
if (Trigger.isInsert) {
Integer recordCount = Trigger.New.size();
// Call a utility method from another class
EmailManager.sendMail('Write your own email address', 'Trailhead Trigger Tutorial',
recordCount + ' contact(s) were inserted.');
}
else if (Trigger.isDelete) {
// Process after delete
}
}
If you do not receive any error message, you can insert a new contact anonymously.
Contact c = new Contact(LastName='Test Contact');
insert c;
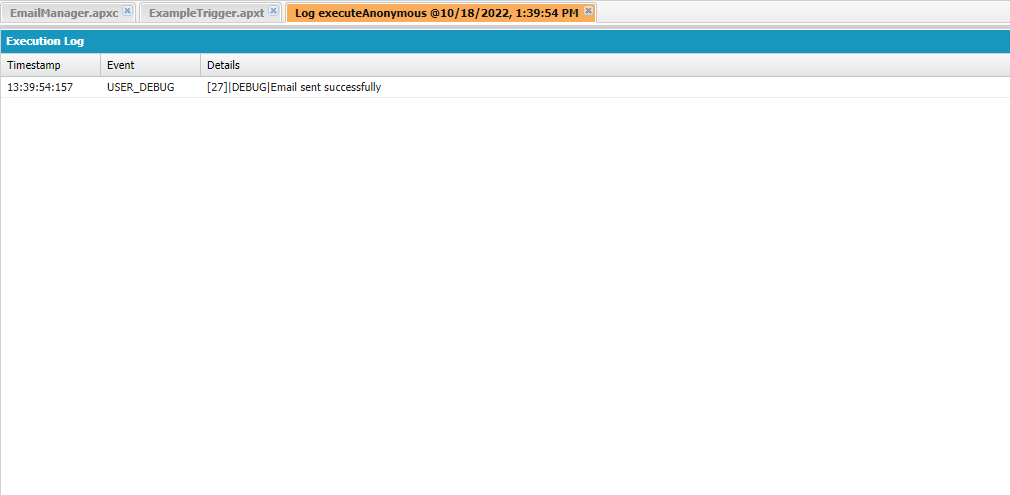
In this practice, we successfully called an apex class method from a trigger.